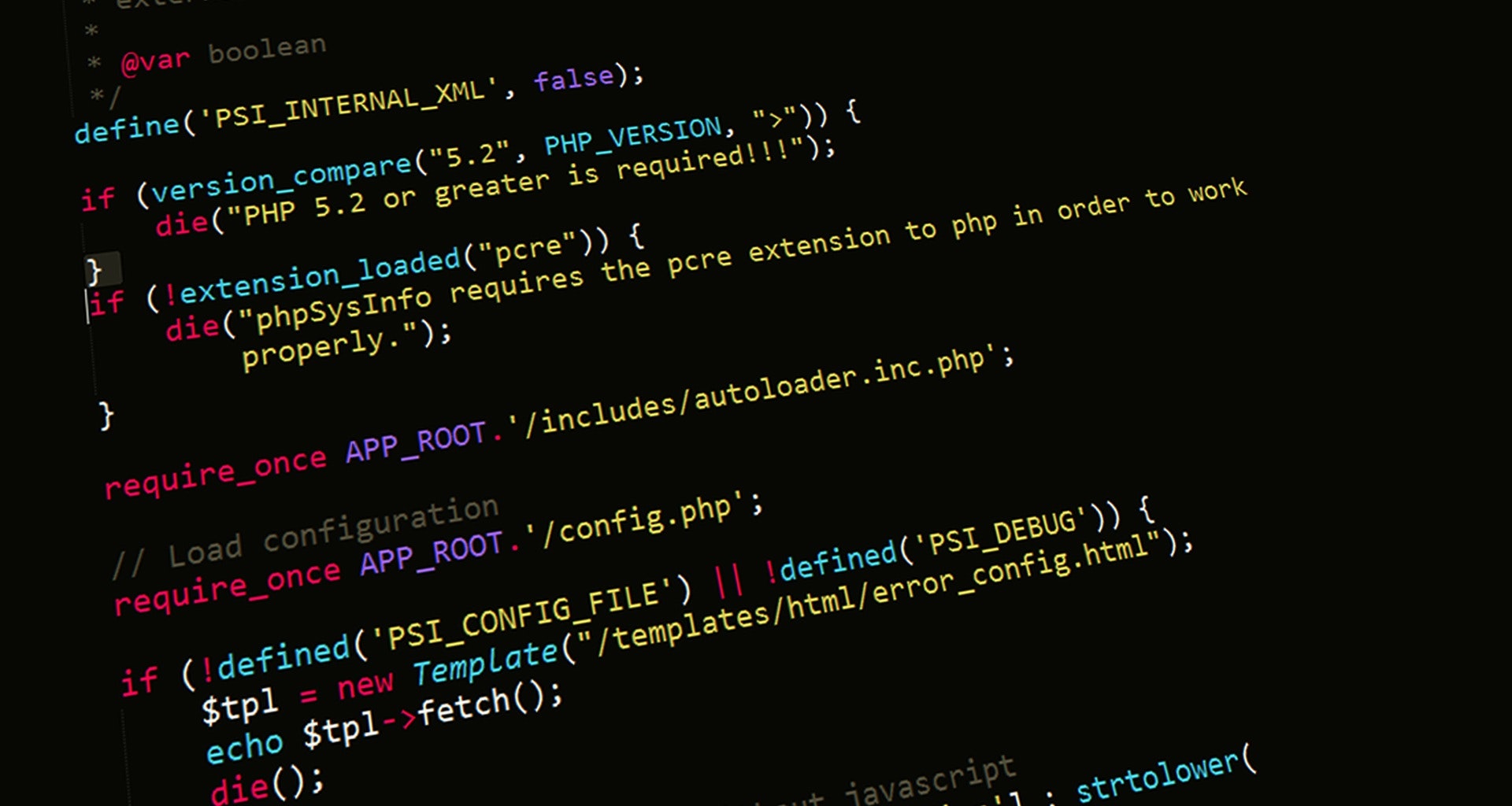
Are you considering building web components for your next project? You may want to use a javascript framework to build these components. One option is a JS framework called Minze.
Minze is a lightweight javascript framework designed for building web components. So let’s take a deeper dive into why Minze may be a good framework for your next project.
First, it’s very lightweight being only 2kb minified and compressed. It’s designed to allow writing components that will be native anywhere, no matter what framework you are already using, for example, Vue, React, or Angular. The main goal is to provide more reusability without hitting the barriers of other frameworks. For example, you can use the same component with both React and Vue without having to use the same syntax. This can help speed up development since you won’t have to re-write any components if you are using multiple frameworks.
Setting up Minze
To set up Minze, you will need to go through a quick installation. Use the following commands.
npm i -g minze #Installs Minze globally
Next, you can initialize a new project by using the following:
npm init minze@latest
You will then be prompted to add a project name.
You will also be asked if you want to use JavaScript or TypeScript. You will need to choose the option that suits you best.
Once you’ve added a name, you can then change directories to that project using the cd command.
After that, you will need to install the dependencies.
npm install
Once those have been installed, you can then build the app by using the following command.
npm run dev
Your project should be running on the following URL: http://localhost:3000/
Understanding the structure of components
Minze will use a specific structure for the components like you would with any other javascript framework. It’s divided into 3 sections, the declaration, HTML, then CSS. The declaration specifies the data management. The HTML section shows how the component’s UI will display on the page. And the CSS will style the component. It’s quite simple!
Here is an example of the code:
import {MinzeElement} from 'minze';
export class MyComponent extends MinzeElement {
//add your attributes and methods here
html = () => {
//add your HTML here
}
css = () => {
//add your CSS here
}
}
Next, let’s take a look at how you can define data. In general, all data can be accessed by attributes. In order to do this, you can use the “this” keyword.
Properties
You can also add properties to components which will act as non-reactive data.
Here is an example of a property being used:
import Minze, { MinzeElement } from 'minze'
class Element extends MinzeElement {
greet = 'Hello World!'
onReady() {
console.log(this.greet) // Hello World!
}
}
Minze.defineAll([Element])
You can see from the above code that “this” was used to declare the property. There are also reactive properties as well. Here is an example of a reactive property in use:
import Minze, { MinzeElement } from 'minze'
class Element extends MinzeElement {
reactive = [
'time',
['name', 'Emmanuel Yusuf'],
['favNum', 6]
]
onReady() {
console.log(
this.time, // null
this.name, // Emmanuel Yusuf
this.favNum // 6
)
}
}
Minze.defineAll([Element])
If you are declaring a reactive property with only a string, it will provide a name without any value. To add the value, you must add it inside a tuple with two values to make the first value the name and the second value which is assigned to it.
Attribute Properties
Unlike the regular properties, attribute properties are dynamic and reactive, allowing you to add the value when the component is declared. The attribute properties are the same as the reactive properties in that they share the same syntax. These property values, however, can be overridden when the specific component is called.
Conclusion
Now you know a little more about Minze, for more information check out their website and see the documentation they provide.
Overall, Minze is designed to help developers that are tasked with converting components from one framework and its syntax to another. It’s a simple solution that can help save a developer’s time when used right.